Have you ever found yourself in a situation where you don’t want to run certain test cases? Maybe the functionality isn’t ready, or the test is temporarily irrelevant, and running it will just waste time. As testers, we all encounter these moments, and thankfully, TestNG has us covered.
In TestNG, skipping or ignoring test cases is straightforward and super useful when managing large test suites. Whether you’re dealing with work-in-progress code, outdated scenarios, or need to temporarily exclude tests for debugging, TestNG makes it easy. In this article, we’ll discuss how to skip test cases at different levels ( method level, class level, and package level) so you can keep your testing process efficient and hassle-free. Let’s dive in!
- Ignoring only a single test method or test case.
- Ignore all cases within a class and its subclasses.
- And lastly, Ignore all cases within a package and its subpackages.
There are two ways to skip a test case –
- Using @Ignore annotation ( can be used at all levels – method, class and package )
- Using enabled attribute of @Test annotation ( mostly used at method level )
In case of a conflict between @Ignore and the enabled attribute for a particular test case. Then, @Ignore is given more priority over the enabled attribute.
Note: There is also a video tutorial at the end of this post. So, if you prefer to have a video walkthrough of the topic, please feel free to check it out.
Ignoring only a single test method or test case
We have placed the @Ignore annotation on the test1 method and @Test(enabled =false) on the test3 method.
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
public class CodekruTest {
@Test @Ignore
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = false)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Running the above class will give you the below output.
Output –
Excecuting test2
PASSED: test2
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
As we can see, only test2 was executed, while test1 and test3 were skipped.
Ignore all cases within a class and its subclasses
This can also be achieved by using the @Ignore annotation. So, if we place @Ignore annotation on a class, then the cases within that class and its subclasses will be ignored. So, let’s see this with an example.
package Test;
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
@Ignore
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = true)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Output –
===============================================
Default test
Tests run: 0, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 0, Failures: 0, Skips: 0
===============================================
[TestNG] No tests found. Nothing was run
So, we can see that none of the cases were executed.
Now. Let’s make a subclass(CodekruTestSubclass) of the above class(CodekruTest), try to execute the subclass test cases, and see what happens.
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
class CodekruTestSubclass extends CodekruTest{
@Test
public void test1() {
System.out.println("Excecuting test1 of subclass");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2 of subclass");
Assert.assertTrue(true);
}
}
@Ignore
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = true)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Output –
===============================================
Default test
Tests run: 0, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 0, Failures: 0, Skips: 0
===============================================
[TestNG] No tests found. Nothing was run
Here, the cases of the subclass weren’t executed because we used @Ignore annotation on the parent class.
Ignore all cases within a package and its subpackages
We can also ignore the test cases in a package and its subpackages. But, we can’t just put the @Ignore annotation at the top of our package, as shown in the below code. Instead, we have to make another package-info.java file and add the package-level annotation.
@Ignore // this is wrong and is throwing an error
package Test;
import org.testng.Assert;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
}
How to create a package-info.java file? Different editors provide different ways; in Eclipse, you can tick the “Create package-info.java” checkbox while creating a new Java package.
- Select New -> Package.
- Now, tick the checkbox package-info.java
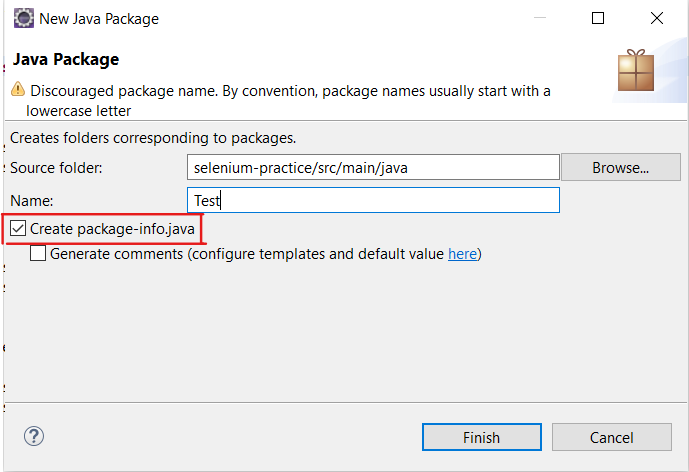
This will create a package-info.java file in the package mentioned above. So, now, we can write @Ignore on the Test package as shown below.
@org.testng.annotations.Ignore
package Test;
So, all cases in this package and its sub-packages will now be ignored.
Video Tutorial
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.